일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | ||||||
2 | 3 | 4 | 5 | 6 | 7 | 8 |
9 | 10 | 11 | 12 | 13 | 14 | 15 |
16 | 17 | 18 | 19 | 20 | 21 | 22 |
23 | 24 | 25 | 26 | 27 | 28 | 29 |
30 | 31 |
- 완전탐색
- Spring
- 실버1
- Floyd
- 스프링
- professional
- 트리의지름
- 최소신장트리
- 최단경로탐색
- 골드3
- backend
- swea
- 그래프
- 순열
- D4
- dp
- 골드5
- 코딩테스트
- 알고리즘
- BOJ
- java
- BFS
- Framework
- 최단경로
- 중복순열
- 1251
- SW역량평가
- SW역량테스트
- 백엔드
- 백준
- Today
- Total
공부 기록장
[SPRING] DI (의존성 주입) 본문
스프링을 시작할 때, 가장 먼저 해 주어야 하는 것이 기본 설정을 세팅하고, 알맞은 곳에 필요한 것들을 주입시키는 것이다.
스프링 빈은 기본적으로 싱글톤으로 만들어진다. 따라서, 컨테이너가 제공하는 모든 빈의 인스턴스는 항상 동일하다.
만약, 컨테이너가 항상 새로운 인스턴스를 반환하게 하고 싶은 경우 scope를 prototype으로 설정해주어야 한다.
스프링 빈 설정은 주로 xml 문서 형태로 기술되어 있는데, 단순하고 사용하기 쉽기 때문이다.
bean 태그를 이용해 pom.xml, root-context.xml, servlet-context.xml 에 필요한 설정들을 넣어준다.
빈을 사용할때 constructor-arg나 property 설정들도 들어있는데,
constructor-arg 태그는 생성자 역할을, property는 객체나 값을 주입 하는 setter 역할을 한다.
전에 만들었던 page의 root-context 설정 파일인데, 주로 DB와 관련 된 설정들이 담겨있다.
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:tx="http://www.springframework.org/schema/tx"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans https://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.3.xsd
http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-4.3.xsd
http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-4.3.xsd">
<!-- Root Context: defines shared resources visible to all other web components -->
<context:component-scan base-package="com.ssafy.guestbook.model, com.ssafy.guestbook.aop" />
<aop:aspectj-autoproxy></aop:aspectj-autoproxy>
<!-- MyBatis-Spring 설정 -->
<bean id="dataSource" class="org.springframework.jndi.JndiObjectFactoryBean">
<property name="jndiName" value="java:comp/env/jdbc/ssafy"></property>
</bean>
<bean id="sqlSessionFactoryBean" class="org.mybatis.spring.SqlSessionFactoryBean">
<property name="dataSource" ref="dataSource"/>
<property name="typeAliasesPackage" value="com.ssafy.guestbook.model"></property>
<property name="mapperLocations" value="classpath:/mapper/*.xml"></property>
</bean>
<bean id="sqlSession" class="org.mybatis.spring.SqlSessionTemplate">
<constructor-arg ref="sqlSessionFactoryBean"></constructor-arg>
</bean>
<bean id="transactionManager" class="org.springframework.jdbc.datasource.DataSourceTransactionManager">
<property name="dataSource" ref="dataSource" />
</bean>
<tx:annotation-driven transaction-manager="transactionManager"/>
</beans>
같은 프로젝트의 servelt-context. 여기는 주로 컨트롤러와 관련된 설정들이 담겨있다.
필요한 부분은 컨트롤러로 연결시켜주고, 컨트롤러로 가는 것을 막아야 하는 부분에서는 막아주는 역할도 한다.
<?xml version="1.0" encoding="UTF-8"?>
<beans:beans xmlns="http://www.springframework.org/schema/mvc"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:beans="http://www.springframework.org/schema/beans"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/mvc https://www.springframework.org/schema/mvc/spring-mvc.xsd
http://www.springframework.org/schema/beans https://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context https://www.springframework.org/schema/context/spring-context.xsd">
<!-- DispatcherServlet Context: defines this servlet's request-processing infrastructure -->
<!-- Enables the Spring MVC @Controller programming model -->
<annotation-driven />
<resources mapping="/img/**" location="/resources/img/"/>
<resources mapping="/css/**" location="/resources/css/"/>
<!-- Resolves views selected for rendering by @Controllers to .jsp resources in the /WEB-INF/views directory -->
<beans:bean class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<beans:property name="prefix" value="/WEB-INF/views/" />
<beans:property name="suffix" value=".jsp" />
</beans:bean>
<context:component-scan base-package="com.ssafy.guestbook.controller, com.ssafy.interceptor" />
<beans:bean id="confirm" class="com.ssafy.interceptor.ConfirmInterceptor"/>
<interceptors>
<interceptor>
<mapping path="/article/write"/>
<mapping path="/article/modify"/>
<mapping path="/article/delete"/>
<beans:ref bean="confirm"/>
</interceptor>
</interceptors>
<!-- fileUpload -->
<beans:bean id="multipartResolver" class="org.springframework.web.multipart.commons.CommonsMultipartResolver">
<beans:property name="defaultEncoding" value="UTF-8"/>
<beans:property name="maxUploadSize" value="52428800"/>
<beans:property name="maxInMemorySize" value="1048576"/>
</beans:bean>
<!-- fileDownload -->
<!-- 다운로드도 링크기 때문에 컨트롤러로 이동. 컨트롤러에서 뷰로 리턴해주는데, view resolver한테 보내지 말고 빈의 이름을 그대로 view로 사용하게 하는 설정. -->
<beans:bean id="fileDownLoadView" class="com.ssafy.guestbook.view.FileDownLoadView"/>
<beans:bean id="fileViewResolver" class="org.springframework.web.servlet.view.BeanNameViewResolver">
<beans:property name="order" value="0" />
</beans:bean>
</beans:beans>
또 다른 기능은 Annotation이 있다.
어플리케이셔느이 규모가 커지고, 빈의 개수가 많아지면 xml 파일을 관리하는 것이 번거로워진다.
따라서 그냥 사용하고자 하는 변수 위에 @Autowired 를 선언해주면 자동으로 값이 매핑되어 들어간다.
이 예제에서는 같은 이름의 타입이 여러개가 있어서 특정시켜준 것이다.
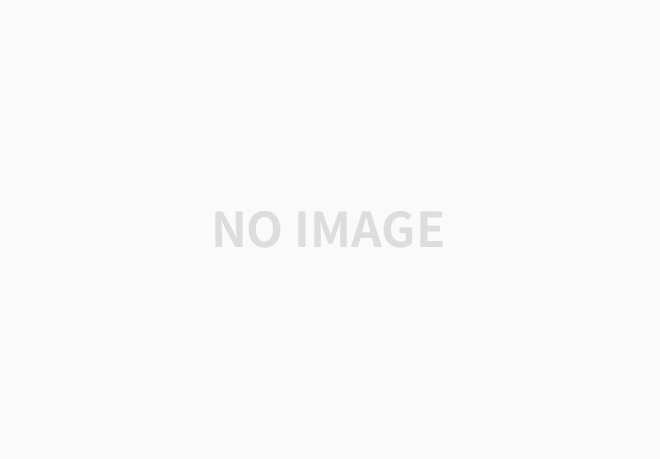
하지만 주의할 점이 있다면, 반드시 component-scan을 설정해주어야 한다. 사용하고자 하는 목적에 따라 servlet-context 파일 속이 될 수도 있고, root-context 파일 속 일 수도있다.
마지막으로 스프링 빈의 생명 주기를 보면,
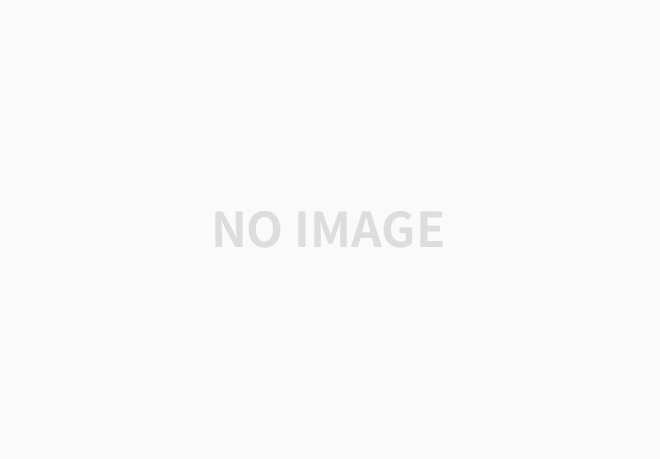
이런 라이프싸이클을 갖고 있는데, 스프링이 알아서 다 해줘서 우리가 굳이 건드릴 필요는 없다.
'웹 > Backend' 카테고리의 다른 글
[SPRING] Spring MVC (0) | 2021.05.15 |
---|---|
[SPRING] 스프링이란? (2) (0) | 2021.05.14 |
[SPRING] 스프링이란? (1) (0) | 2021.04.27 |
[Backend] MVC 패턴 (0) | 2021.04.05 |
JSP 정리 (0) | 2021.04.04 |